Optimize Your Laravel Queries: Fix the N+1 Query Issue
Improve your Laravel application's performance by using eager loading instead of inefficient queries inside loops
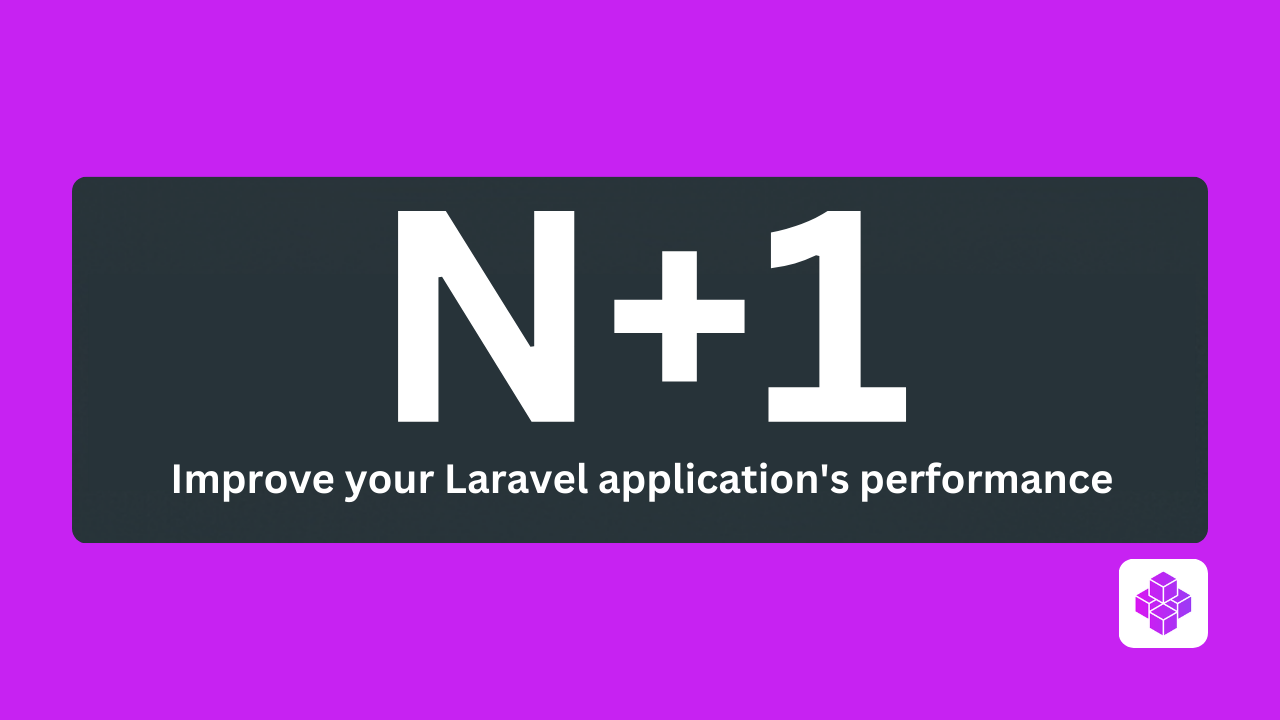
Understanding the N+1 Query Problem
A common performance issue in Laravel applications is the N+1 query problem. This occurs when a query retrieves a list of models, and then additional queries are executed to fetch related data for each model individually.
The Problem: Running Queries Inside a Loop
$users = User::all(); foreach ($users as $user) { $user->posts = Post::where('user_id', $user->id)->get(); // Inefficient }
If there are 100 users, this approach results in 101 queries—one for retrieving users and 100 more for fetching posts. This drastically slows down performance and increases database load.
The Solution: Use Eager Loading
Eager loading allows Laravel to retrieve related models in a single query, preventing unnecessary database calls.
$users = User::with('posts')->get(); foreach ($users as $user) { $user->posts; // Already loaded, no extra queries }
Why Eager Loading is Better
- Reduces the number of queries from N+1 to just 1 or 2.
- Improves performance by minimizing database calls.
- Simplifies and cleans up code, making it easier to maintain.
Alternative Ways to Prevent N+1 Issues
1. Use the $with
Property in Models
Instead of manually calling with()
, you can define related models to be eager-loaded automatically every time you query the model.
class User extends Model { protected $with = ['posts']; }
Pros:
- Ensures the related models are always loaded.
- Eliminates the need to manually specify eager loading each time.
Cons:
- Increases query complexity, even when the related models are not needed.
- May load unnecessary data, affecting memory usage.
2. Use preventLazyLoading
to Detect N+1 Queries
Since Laravel 9, you can enable preventLazyLoading()
in development mode to catch accidental N+1 queries before they impact performance.
use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\LazyLoadingViolationException; Model::preventLazyLoading();
Now, if an undeclared relationship is accessed without eager loading, Laravel will throw an exception:
LazyLoadingViolationException: Attempted to access [posts] without eager loading
Pros:
- Helps detect and prevent N+1 query issues during development.
- Encourages developers to use eager loading properly.
Cons:
- Throws exceptions in development, which may be disruptive if not properly handled.
- Must be disabled in production to avoid breaking the application.
3. On-Query Eager Loading with Constraints
You can apply constraints directly within the with()
method to eager load specific subsets of related data.
$users = User::with(['posts' => function ($query) { $query->where('status', 'published'); }])->get();
This approach retrieves users along with only their published posts, optimizing data retrieval.
Pros:
- Fetches only the necessary related data, reducing memory usage.
- Allows for fine-grained control over the eager loading process.
Cons:
- Adds complexity to the query structure.
- Requires careful management to ensure constraints are correctly applied.
Additional Tips to Improve Query Performance
1. Use load()
for Eager Loading After Fetching Models
If you have already retrieved models and later realize you need their relationships, you can eager load them with load()
.
$users = User::all(); $users->load('posts');
This avoids re-fetching the users while still optimizing queries for related data.
2. Use select()
to Load Only Necessary Columns
If you don’t need all columns, optimize your queries by selecting only the necessary fields.
$users = User::with('posts:id,user_id,title')->get();
This retrieves only id
, user_id
, and title
from the posts
table, reducing memory usage.
Conclusion
To avoid the N+1 query issue in Laravel:
- Always use
with()
when fetching related models. - Use the
$with
property if relationships should always be loaded. - Enable
preventLazyLoading()
to detect N+1 problems in development. - Apply constraints within the
with()
method for on-query eager loading. - Use
load()
if relationships need to be fetched after retrieving models. - Optimize queries by selecting only required columns.
By applying these techniques, you can ensure your Laravel application runs efficiently, reducing unnecessary database queries while keeping the codebase clean and scalable.